XML Key Management Service (XKMS)
Available since CXF 2.7.7.
Use case
CXF uses asymmetric algorithms for different purposes: encryption of symmetric keys and payloads, signing security tokens and messages, proof of possession, etc.
Normally the public keys (in the form of X509 certificates) are stored in java keystores.
For example, if the sender encrypts the message payload sending to the receiver, he should have access to the receiver certificate saved in the local keystore.
The sender uses this certificate for message encryption and receiver decrypts the request with the corresponding private key:
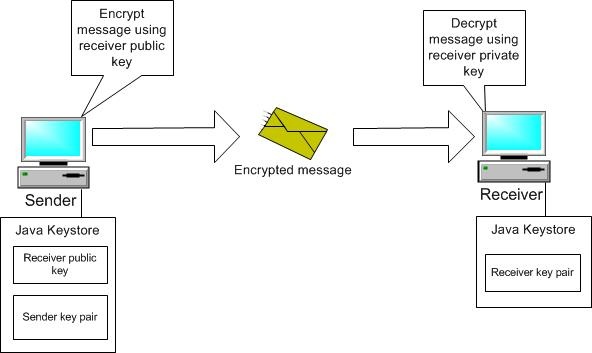
Seems to be OK? Imagine now that you have a production environment with 100 different clients of this service and the service certificate is expired. You should reissue and replace the certificate in ALL client keystores! Even more, if keystores are packaged into war files or OSGi bundles – they should be unpackaged and updated. Not really acceptable for enterprise environments.
Therefore large service landscapes support central certificates management. It means that X509 certificates are not stored locally in keystores, but are provided and administrated centrally.
Normally it is a responsibility of Public Key Infrastructure (PKI) established in the organization. PKI is responsible to create, manage, store, distribute, synchronize and revoke public certificates and certification authorities (CAs).
XKMS Specification
W3C specifies a protocol to distribute and register public keys, certificates and CAs that can be used for XML-based cryptography, including signature and encryption: XML Key Management Specification (XKMS 2.0).
The XKMS Specification comprises two parts – the XML Key Information Service Specification (XKISS) describing the runtime aspects of key lookup and certificate validation, and the XML Key Registration Service Specification (XKRSS) describing the administrative aspects of registering, renewing, revoking and recovering certificates.
The XKMS Service implements both parts of specification.
The XKMS SOAP interface can be used as a standard frontend to access the Public Key Infrastructure (PKI). Using XKMS message encryption scenario, the message encryption picture will change in the following way:
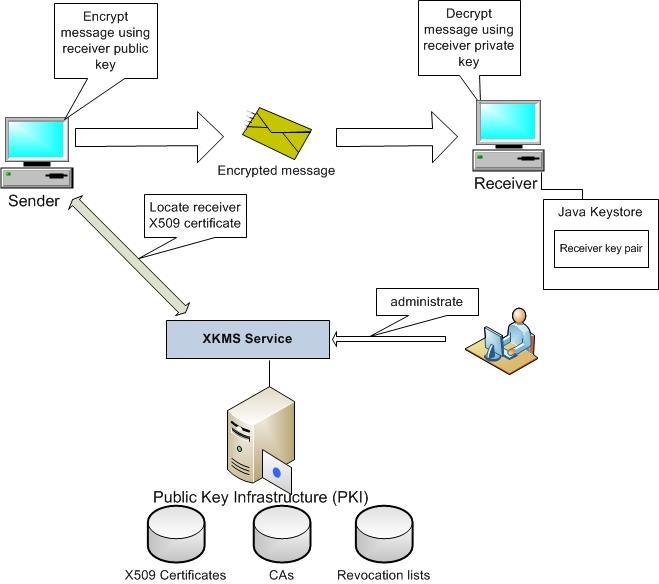
Receiver X509 certificate is not saved into sender's local keystore anymore. Instead, certificate is stored into central PKI and can be located, validated and administrated using standard XKMS interface. This essentially improves the control on certificates in large services landscape.
Administrator can update, renew and revoke certificates, manage certification authorities and revocation lists.
Integrating the XKMS client into the CXF runtime
The XKMS client can be integrated into CXF and WSS4J in pretty elegant way using a custom Crypto provider implementation. In this case, the XKMS service will be automatically invoked when WSS4J asks for the certificates or validates them. Details are described in this blog. A basic XKMS implementation of WSS4J Crypto interface is available in XKMS Client component (XKMSCryptoProvider and XKMSCryptoProviderFactory). Implementation uses Ehcache to cache certificates received from XKMS service.
XKMS Service Design
Internal structure of XKMS service is represented in the following figure:
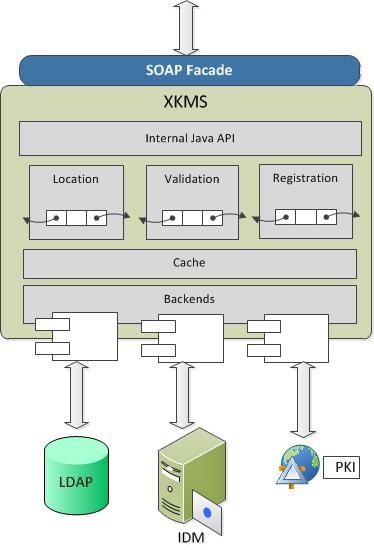
The XKMS Service exposes a SOAP interface specified in XKMS 2.0.
The XKMS implementation realizes chain of responsibility design pattern .
Each XKMS operation defines a handler interface and provides one or more implementations of this interface. Handler implementations are connected into a chain.
Operation implementation invokes handlers one after another from the pre-configured chain until either all handlers will be processed or a critical error will occur.
This design makes the XKMS internal implementation quite flexible: it is easy to add/remove handlers, change their order, introduce handlers supporting new backends, etc.
For example, a certificate can be searched firstly in the LDAP repository by LDAP lookup handler and, if it is not found there, additionally looked for in a remote PKI using an appropriate lookup handler. Validation operation logic is organized in a chain is well: first validation handler checks format and expiry date of the X509 certificate, next one checks the certificate trust chain.
Currently the XKMS Service supports simple file based and LDAP backends.
Sample spring configuration of XKMS handlers looks like:
xsi:schemaLocation="
http:
http:
http:
http:
http:
http:
http:
http:
<bean id= "dateValidator" class = "org.apache.cxf.xkms.x509.validator.DateValidator" />
<bean id= "trustedAuthorityValidator"
class = "org.apache.cxf.xkms.x509.validator.TrustedAuthorityValidator" >
<constructor-arg ref= "certificateRepo" />
</bean>
<bean id= "x509Locator" class = "org.apache.cxf.xkms.x509.handlers.X509Locator" >
<constructor-arg ref= "certificateRepo" />
</bean>
<bean id= "x509Register"
class = "org.apache.cxf.xkms.x509.handlers.x509Register" >
<constructor-arg ref= "certificateRepo" />
</bean>
<!-- LDAP based implementation -->
<bean id= "certificateRepo"
class = "org.apache.cxf.xkms.x509.repo.ldap.LdapCertificateRepo" >
<constructor-arg ref= "ldapSearch" />
<constructor-arg ref= "ldapSchemaConfig" />
<constructor-arg value= "dc=example,dc=com" />
</bean>
<bean id= "ldapSearch" class = "org.apache.cxf.xkms.x509.repo.ldap.LdapSearch" >
<constructor-arg value= "cn=Directory Manager,dc=example,dc=com" />
<constructor-arg value= "test" />
<constructor-arg value= "2" />
</bean>
<bean id= "ldapSchemaConfig" class = "org.apache.cxf.xkms.x509.repo.ldap.LdapSchemaConfig" >
<property name= "certObjectClass" value= "inetOrgPerson" />
<property name= "attrUID" value= "uid" />
<property name= "attrIssuerID" value= "manager" />
<property name= "attrSerialNumber" value= "employeeNumber" />
<property name= "attrCrtBinary" value= "userCertificate;binary" />
<property name= "constAttrNamesCSV" value= "sn" />
<property name= "constAttrValuesCSV" value= "X509 certificate" />
<property name= "serviceCertRDNTemplate" value= "cn=%s,ou=services" />
<property name= "serviceCertUIDTemplate" value= "cn=%s" />
<property name= "trustedAuthorityFilter" value= "(&(objectClass=inetOrgPerson)(ou:dn:=CAs))" />
<property name= "intermediateFilter" value= "(objectClass=inetOrgPerson)" />
</bean>
<!-- File based implementation -->
<!-- bean id= "certificateRepo"
class = "org.apache.cxf.xkms.x509.repo.file.FileCertificateRepo" >
<constructor-arg value= "../conf/certs" />
</bean-->
</beans>
|
The dateValidator and trustedAuthorityValidator beans are implementations of the Validator interface for date and trusted chain validation.
x509Locator and x509Register are implementations of Locator and Register interfaces for X509 certificates.
certificateRepo is the repository implementation for LDAP backend. LdapSearch and LdapSchemaConfig contain LDAP configuration described in the following table:
Property | Sample Value | Description |
---|
ldapServerConfig arguments | | URL, baseDN and credentials of LDAP Server |
certObjectClass | inetOrgPerson | LDAP object class used to store certificates |
attrUID | uid | Attribute containing X509 subject DN |
attrIssuerID | manager | LDAP attribute containing X509 issuer DN |
attrSerialNumber | employeeNumber | LDAP attribute containing X509 serial number |
attrEndpoint | labeledURI | LDAP attribute containing service endpoint (used in case of endpoint based lookup) |
attrCrtBinary | userCertificate | LDAP attribute containing X509 certificate content |
constAttrNamesCSV | sn | Comma separated list of mandatory LDAP attributes |
constAttrValuesCSV | X509 certificate | Comma separated list of mandatory LDAP attributes values |
serviceCertRDNTemplate | cn=%s,ou=services | Relative distinguished name for service certificates |
serviceCertUIDTemplate | cn=%s | Template to transform service QName to DN for storing into attrUID |
trustedAuthorityFilter | (&(objectClass=inetOrgPerson)(ou:dn:=CAs)) | Filter to determine trusted CAs for trusted chain validation |
intermediateFilter | (objectClass=inetOrgPerson) | Filter to determine intermediate certificates for trusted chain validation |
Supported certificates types.
XKMS distinguishes between the following types of X509 certificates:
Type | Description |
---|
User | Normal user X509 certificate |
Service | Certificate identifies service. Required application "urn:apache:cxf:service:soap" by lookup and registration. Identified as {SERVICE_ NAMESPACE}SERVICE_NAME |
Trusted CA | CAs used as trusted anchor by certificates validations. Trusted CAs can be retrieved using trustedAuthorityFilter property |
XKMS service endpoint is configured in the following way:
<bean id= "xkmsProviderBean" class = "org.apache.cxf.xkms.service.XKMSService" >
<property name= "validators" >
<list>
<ref bean= "dateValidator" />
<ref bean= "trustedAuthorityValidator" />
</list>
</property>
<property name= "locators" >
<list>
<ref bean= "x509Locator" />
</list>
</property>
<property name= "keyRegisterHandlers" >
<list>
<ref bean= "x509Register" />
</list>
</property>
</bean>
<jaxws:endpoint id= "XKMSService"
serviceName= "serviceNamespace:XKMSService" endpointName= "serviceNamespace:XKMSPort"
implementor= "#xkmsProviderBean" address= "/XKMS" >
</jaxws:endpoint>
|
Input and output data formats are specified in XML Key Management Service Specification Version 2.0 (see XKMS 2.0). The XKMS service supports only a subset of the specified requests and responses.
Restrictions of formats for request and responses are described in the following table:
Element XPath | Supporting values | Description |
---|
RootElement/QueryKeyBinding/UseKeyWith@Application | urn:ietf:rfc:2459 | Application specifies X509 SubjectDN in Identifier attribute. Used for normal users certificates |
urn:apache:cxf:service:name | Application specifies service name in Identifier attribute as {SERVICE_ NAMESPACE}SERVICE_NAME. Used for service certificates |
urn:apache:cxf:service:endpoint | Application specifies service endpoint in Identifier attribute |
RootElement/QueryKeyBinding/UseKeyWith@Identifier | - X509 Subject DN;
- Service name as {SERVICE_ NAMESPACE}SERVICE_NAME
- Service endpoint
| Depending on Application attribute public key is identified as X509 Subject DN or Service nameservice certificates |
RootElement/UnverifiedKeyBinding/KeyInfo | X509Data/X509Certificate | Only X509Data with X509Certificate is supported |
Error Handling
Success and Fault Response formats are specified in XKMS 2.0. Error conditions in XKMS service are reported using ResultMajor and ResultMinor attributes in the root response element.
The XKMS Service uses the following values for response codes:
ResultMajor
Value | Description |
---|
Success | The operation succeeded. |
Receiver | An error occurred at the receiver. |
Sender | An error occurred that was due to the message sent by the sender. |
ResultMinor
Value | Description |
---|
Failure | The service attempted to perform the request but the operation failed. |
NoMatch | No match was found for the search prototype provided. |
TooManyResponses | The request resulted in the number of responses that exceeded limit determined by the service. |
TimeInstantNotSupported | The receiver has refused the operation because it does not support the TimeInstant element. |
Deployment
The XKMS Service can be deployed into web and OSGi containers. The Service implementation was tested with Tomcat and Karaf.
Sample Requests and Responses
Sample request for Locate operation:
<soap:Body>
<ns2:QueryKeyBinding>
<ns2:UseKeyWith Application= "urn:ietf:rfc:2459"
Identifier="EMAILADDRESS=client @client .com, CN=www.client.com, OU=IT Department,
O=Sample Client -- NOT FOR PRODUCTION, L=Niagara Falls, ST=New York, C=US" />
</ns2:QueryKeyBinding>
</ns2:LocateRequest>
</soap:Body>
</soap:Envelope>
|
Sample response for Locate operation:
<soap:Body>
RequestId= "I047257513d19456687e6b4f4a2a72606" Id= "I0758390284847918129574923948"
<ns2:UnverifiedKeyBinding>
<ns4:KeyInfo>
<ns4:X509Data>
<ns4:X509Certificate>… </ns4:X509Certificate>
</ns4:X509Data>
</ns4:KeyInfo>
</ns2:UnverifiedKeyBinding>
</ns2:LocateResult>
</soap:Body>
</soap:Envelope>
|
Sample error message:
<soap:Body>
RequestId= "I047257513d19456687e6b4f4a2a72606" Id= "I0758390284847918129574923948"
<ns2:MessageExtension xsi:type= "ns5:resultDetails"
<Details>Search certificates failure: Application
identifier not supported</Details>
</ns2:MessageExtension>
</ns2:LocateResult>
</soap:Body>
</soap:Envelope>
|
Current restrictions and ToDos
- only X509 certificates are supported as keys;
- only LDAP and File based backends are supported;
- more integration tests are required