This page summarizes an experience and use cases of implementing a new custom CXF transport.
Use Cases
Basically there are two main use cases for implementing a new CXF transport:
- Providing a new physical protocol not yet supported by CXF (udp or ftp, for example). Some such cases can be solved by using integration with a corresponding Camel component, but if no such component is available or workable creating a new custom CXF transport should be considered.
- Supporting tight integration with another framework (like JBI or Camel). In this case integration would be kept transparent for CXF applications - they would just speak directly with the target framework on the transport level. Here, the Transport implementation would be responsible for converting and transferring CXF exchange, messages and faults to target framework.
Presently the CXF distribution provides a transport implementation for the following protocols: HTTP(S), JBI, JMS and Local(inside one JVM). Camel additionally implements a CXF transport for Camel exchanges.
Architecture and Design
The transport functionality is based on two fundamental definitions: conduit and destination. Conduits are responsible for sending a message to recipients and destinations for receiving a message from the sender. In order to send a response, a destination needs its own back-channel conduit (in case of request-response communication). Conduits and destinations are created by a TransportFactory. CXF selects the correct TransportFactory based on the transport URL. SOAP is also considered a high level transport and has its own conduit and destination in CXF.
To send a message into a physical channel, the conduit should access the message context. Normal practice in this case would be to use a subclass of OutputStream extending CachedOutputStream. The custom stream will be fed the message and provided the possibility to access context in streaming or buffered form depending on the transport requirements. CachedOutputStream is configured to keep message in memory only up to a predefined size. If this size is exceeded, the message is swapped to disk.
A class diagram of TransportFactory, Conduit, Destination and OutputStream is shown below:
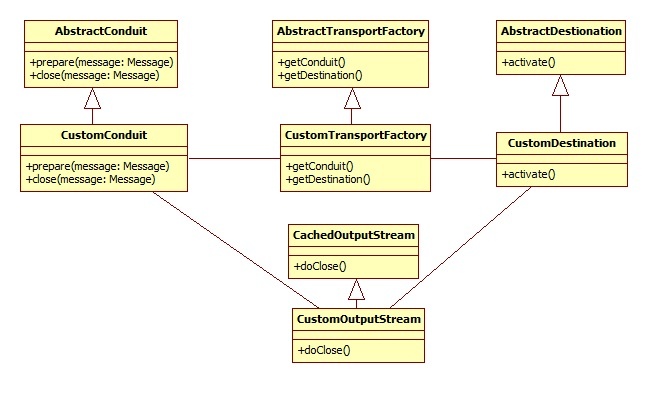
How it Works
Interaction between JAX-WS client and service using CXF transport is represented in the following figure:
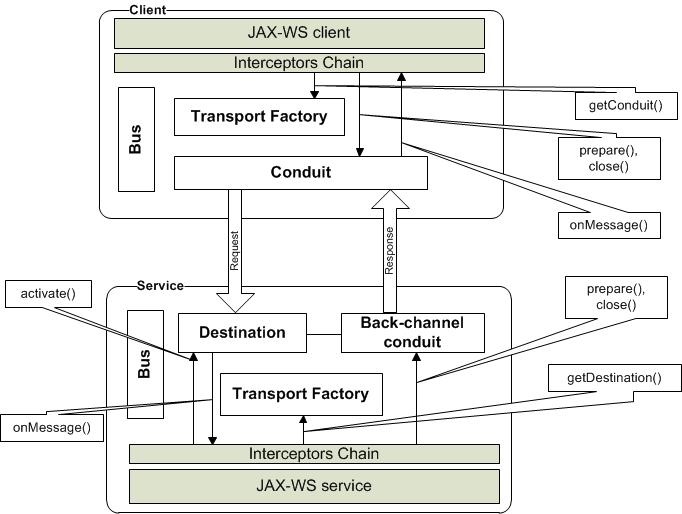
Simplified Client Workflow:
- Step1: The JAX-WS client invokes a service, in this manner for example:
URL wsdlURL = this.getClass().getResource("/HelloWorld.wsdl");
HelloWorldService service = new HelloWorldService(wsdlURL, SERVICE_NAME);
HelloWorld hw = service.getHelloWorldPort();
String result = hw.sayHi(TEST_REQUEST); }}
- Step2: The CXF runtime selects the correct TransportFactory based on some criteria (described below)
- Step3: The CXF runtime calls TransportFactory.getConduit() method to obtain the conduit
- Step4: The CXF runtime invokes Conduit.prepare() and passes the outgoing message as an argument
- Step5: Conduit sets own OutputStream (normally extended CachedOutputStream) as the outgoing message content
- Step6: CXF runtime processes outgoing message, calls the interceptor chain and writes outgoing message to conduit’s OutputStream stream. Messaging in CXF is stream-oriented; therefore the message normally is proceed and sent not as one bunch, but as a stream. The last bytes of the message can still be in processing by sender, but the first already sent to recipient. Basically it is responsibility of Conduit how to send the message: using streaming or collecting the whole message and send it at once
- Step7: When CXF runtime completely proceeded outgoing message, it invokes Conduit.close(Message) method. It means that the message is completely written into OutputStream. Correspondingly, OutputStream.doClose() method will be called
- Step8: In the doClose() method, Conduit sends the rest of the message (or whole message) to recipient
- Step9: In case of one-way communication exchange will be closed. Skip to Step 14
- Step10: In case of request-response communication, the conduit will wait for the service response in a synchronous or asynchronous manner
- Step11: If a successful response is received, the conduit creates a new message, sets its context and puts it as In-Message in the exchange as an incoming message
- Step12: If a fault is instead received, the Conduit creates a new Message, sets its context and places it as a fault message in exchange as in-fault message
- Step13: Conduit notifies incomingObserver (that is ClientImpl object) about the response using incomingObserver.onMessage() call
- Step14: Conduit implementation decreases the reference count with the network connection, potentially closing the connection if the count is zero
- Step15: The JAX-WS client code receives the response in sync or async style
Simplified Service Workflow:
- Step1: JAX-WS service is registered for example in this way:
HelloWorldImpl serverImpl = new HelloWorldImpl();
Endpoint.publish("udp://localhost:9000/hello", serverImpl);
- Step2: The CXF runtime selects correct TransportFactory based on some criteria (described below)
- Step3: The CXF runtime calls TransportFactory.getDestination() method to obtain the destination
- Step4: As soon as the CXF runtime activates the endpoint (adds a listener, etc) the Destination.activate() method will be automatically invoked
- Step5: The implementation of Destination.activate() normally opens network transport connections and listens to incoming requests
- Step6: When a request comes, the destination creates a message, sets the content and notifies message observer (that is ChainInitializationObserver object) via incomingObserver.onMessage() about request. Message content is saved as a stream; therefore runtime and business logic can start processing even not completely received message. Normally an incoming connection is saved in a correlation map to be extracted for the sending of appropriate response
- Step7: The business service implementation will be called with the request message in stream form. In case of one-way communication the exchange is now finished. In case of request-response, the business implementation either returns a response or throws a fault exception
- Step8: The CXF Runtime requests a back-channel conduit from the destination via Destination.getInbuiltBackChannel()
- Step9: The Back-channel conduit's prepare() method will be called with a response message as argument
- Step10: Back-channel conduit sets its own OutputStream as a message context
- Step11: CXF runtime processes the response message, calls the interceptor chain and invokes Conduit.close(Message) for the response message
- Step12. Finally OutputStream.doClose() method for the response message is invoked
- Step13: In doClose() method the OutputStream class has access to the marshaled response message and will send this message through the network as a response to the client. In case of streaming, the part of the message can be already sent to the network at this time, and Conduit just sends the last part and closes the sending. Normally incoming connection for specified protocol is cached and created only if necessary
Registration of Transport Factory
There are two ways to register a transport factory: programmatically or via Spring configuration.
To register transport factory programmatically it is necessary to execute the following code:
Bus bus = BusFactory.getThreadDefaultBus();
DestinationFactoryManagerImpl dfm = bus.getExtension(DestinationFactoryManagerImpl.class);
CustomTransportFactory customTransport = new CustomTransportFactory();
dfm.registerDestinationFactory(TRANSPORT_IDENTIFIER, customTransport);
ConduitInitiatorManager extension = bus.getExtension(ConduitInitiatorManager.class);
extension.registerConduitInitiator(TRANSPORT_IDENTIFIER, customTransport);
TRANSPORT_IDENTIFIER is unique transport id (normally in form “http://apache.org/transports/PROTOCOL_PREFIX”).
For Spring configuration, the following could be used instead:
<bean class="org.company.cxf.transport.CustomTransportFactory"
lazy-init="false">
<property name="transportIds">
<list>
<value>TRANSPORT_IDENTIFIER</value>
</list>
</property>
</bean>
TransportFactory selection
As far as binding TransportFactory is found, CXF looking for protocol TransportFactory responsible for physical network communication. In this case important is method TransportFactory.getUriPrefixes(). This method returns list of protocol prefixes supported by this TransportFactory.
When CXF client or service try to communicate using URL with specified protocol prefix (http://, https://, jms://, local://), CXF looks into registered transport factories map and gets the right one for this prefix. If no TransportFactory for this protocol is found, CXF throws corresponded exception.
Client configuration:
<jaxws:client id="FlightReservationClient"
xmlns:serviceNamespace="http://www.apache.org/cxf/samples/FlightReservation"
serviceClass="org.apache.cxf.samples.flightreservation.FlightReservation"
serviceName="serviceNamespace:FlightReservationService" endpointName="serviceNamespace:FlightReservationSOAP">
address="http://localhost:8040/services/FlightReservationService">
</jaxws:client>
…
TransportFactory class:
…
private static final Set<String> URI_PREFIXES = new HashSet<String>();
static {
URI_PREFIXES.add("http://");
URI_PREFIXES.add("https:");
}
public Set<String> getUriPrefixes() {
return URI_PREFIXES;
}
Conduit and Destination Lifecycle
Destinations are normally created by service on startup and released by shutdown. Conduits can be either recreated for each request or cached based on endpoint information for whole client life time. Clients can make concurrent calls to endpoints using different protocols and bound them to different conduits.
Concurrency Aspects
Conduit and destination objects can by concurrently accessed by multiple threads. Implementations should care about thread safety of the class.
Streaming
It is strongly recommended to don’t break streaming in Conduit and Destination implementations, if physical protocol supports it. CXF is completely streaming oriented – it causes high performance and scalability.
References
What is the start point to understand the CXF transport layer and implement own transport? It makes sense to read CXF documentation CXF transports overview and analyze source code of existing CXF transports (Local and JMS once are more straightforward). They are located into packages: org.apache.cxf.transport.local and org.apache.cxf.transport.jms correspondingly.