LogBrowser
This feature is available from 2.3.0 only, as part of the cxf-rt-management-web component
LogBrowser is log entries viewer. It is rich internet application which can be embedded in your web application. Configure what log entries you would like to see and view them in your favorite browser. Anytime, anywhere without using console. Let's make brief overview how to configure LogBrowser inside your application.
Screenshots
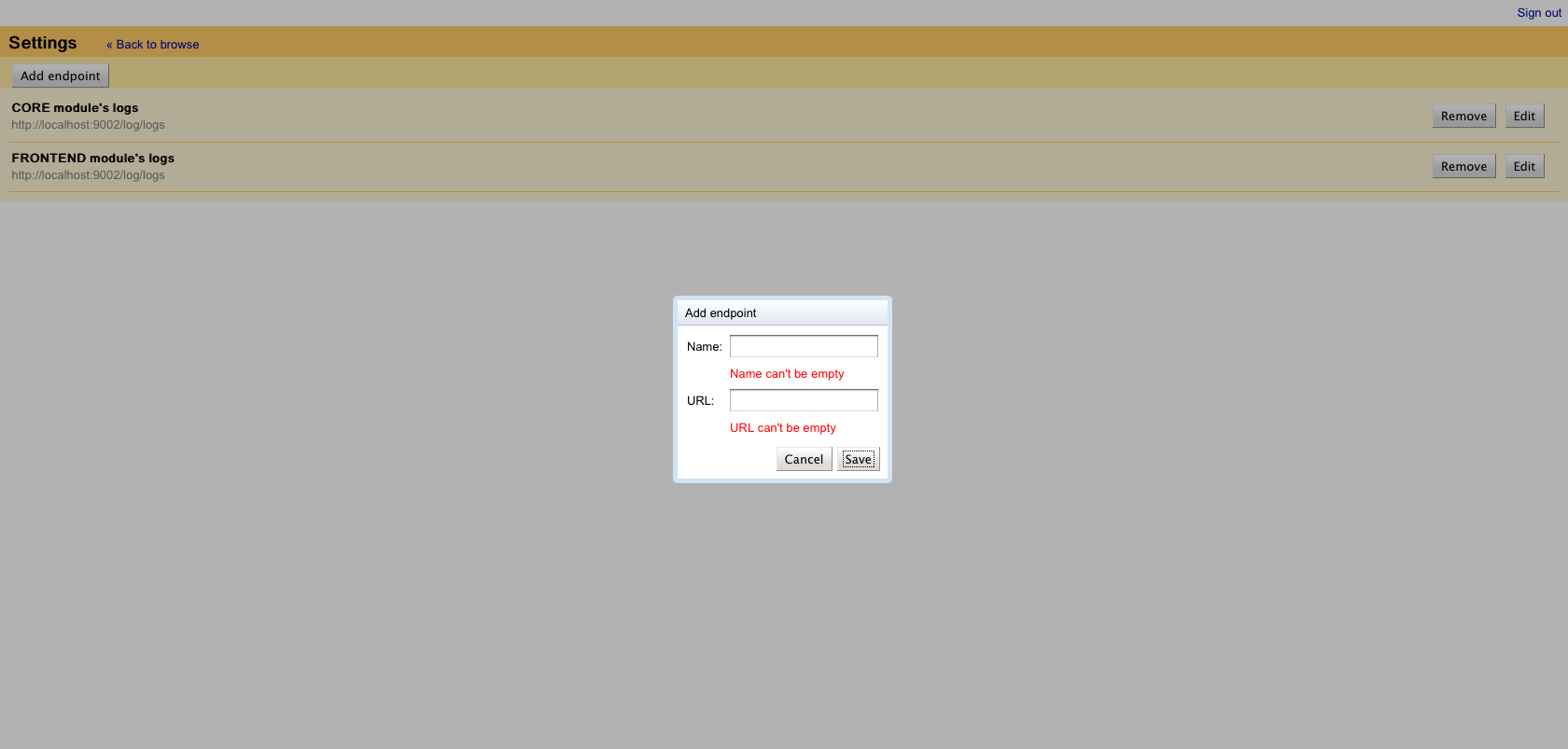
Configuring LogBrowser application in container without Spring
Step 1
Add cxf-rt-management-web
dependency to your Maven project (if you don't use Maven as management tool, omit this step and add cxf-rt-management-web
library manually):
<project>
[...]
<dependencies>
[...]
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-management-web</artifactId>
<version>2.3.0</version>
</dependency>
[...]
</dependencies>
[...]
</project>
Step 2
Add new class to your project, LogBrowserApp
. Add content from sample below.
package yourpackage;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
import javax.ws.rs.core.Application;
import javax.ws.rs.ext.Provider;
import org.apache.cxf.jaxrs.provider.AtomEntryProvider;
import org.apache.cxf.jaxrs.provider.AtomFeedProvider;
import org.apache.cxf.management.web.logging.atom.AtomPullServer;
import org.apache.cxf.management.web.logging.logbrowser.bootstrapping.BootstrapStorage;
import org.apache.cxf.management.web.logging.logbrowser.bootstrapping.SimpleAuthenticationFilter;
import org.apache.cxf.management.web.logging.logbrowser.bootstrapping.SimpleXMLSettingsStorage;
@Provider
public class LogBrowserApp extends Application {
Set<Object> classes = new HashSet<Object>();
static {
AtomPullServer aps = new AtomPullServer();
aps.setLoggers("yourpackage:INFO");
aps.init();
classes.add(aps);
classes.add(new AtomFeedProvider());
classes.add(new AtomEntryProvider());
classes.add(new BootstrapStorage(new SimpleXMLSettingsStorage()));
classes.add(new SimpleAuthenticationFilter(new HashMap<String, String>() {
{
// add all required users by specified username and password, for example: put("admin", "admin");
}
}));
classes.add(new BootstrapStorage.StaticFileProvider());
classes.add(new BootstrapStorage.SettingsProvider());
}
@Override
public Set<Class<?>> getClasses() {
return Collections.emptySet();
}
@Override
public Set<Object> getSingletons() {
return classes;
}
}
We have to modify newly created class a little bit:
- Go to line 1. Replace
yourpackage
phrase with a valid package. - Go to line 23. Configure which log entries should be caught. If you would like to catch all log entries related with your application at the INFO level, simply replace
yourpackage
phrase with your application's package. For more information how to configure custom loggers go to AtomPullServer description. - Go to line 32. Add all required users. Keep in mind that each user has his own independent configuration.
Step 3
Add new servlet definition to your web.xml
file, like in sample below:
<web-app>
[...]
<servlet>
<servlet-name>LogBrowserApp</servlet-name>
<display-name>LogBrowserApp</display-name>
<servlet-class>
org.apache.cxf.jaxrs.servlet.CXFNonSpringJaxrsServlet
</servlet-class>
<init-param>
<param-name>javax.ws.rs.Application</param-name>
<param-value>yourpackage.LogBrowserApp</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>LogBrowserApp</servlet-name>
<url-pattern>/log/*</url-pattern>
</servlet-mapping>
[...]
</web-app>
Go to line 13 and replace yourpackage
phrase with a valid package.
Step 4
Rebuild and run your application.
Step 5
Open browser and go to URL:
http://localhost:9002/log/bootstrapstorage/resources/LogBrowser.html
Step 6
Add new endpoint with URL:
http://localhost:9002/log/logs
Congratulation. Now you can easily view log enties using LogBrowser.